Generate a QR Code
Creates a QR code image. You must use a PUBLIC KEY for this request.
Request
curl "https://api-eu1.stannp.com/v1/qrcode/create" \
-u {API_KEY}: \
-d "size=300" \
-d "data=hello+world"
Response
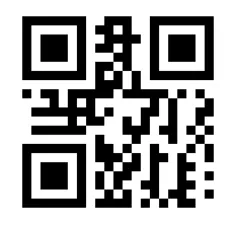
Merge PDF files
Merge multiple PDF files into a single file.
Request
curl "https://api-eu1.stannp.com/v1/pdf/merge" \
-u {API_KEY}: \
-d "files[0]=https://file1.pdf" \
-d "files[1]=https://file2.pdf"
Response
{ "success": true, "data": "https:\/\/merged-file.pdf" }
Get templates
Get the templates created on your account.
Request
curl "https://api-eu1.stannp.com/v1/templates/list" \
-u {API_KEY}: \
Response
{ "success": true, "data": [ { "id": "0", "account_id": "0", "template_name": "Template name 1", "image": "", "created": "2023-09-06 12:37:21", "updated": "2023-11-01 09:53:20", "size": "US-letter", "page_count": "1", "duplex": "1", "back_image": null, "template": "", "is_public": "1", "is_template": "1", "version": "3", "is_hidden": "1", "shared": "0", "user_id": "0", "tags": "", "versions": "3" }, { "id": "0", "account_id": "0", "template_name": "Template name 2", "image": "", "created": "2023-09-06 11:44:37", "updated": "2023-11-01 09:52:50", "size": "4X6", "page_count": "1", "duplex": "1", "back_image": null, "template": "", "is_public": "1", "is_template": "1", "version": "3", "is_hidden": "1", "shared": "0", "user_id": "0", "tags": "", "versions": "2" } ] }