Generate a QR Code
Creates a QR code image. You must use a PUBLIC KEY for this request.
Request
curl "https://api-us1.stannp.com/v1/qrcode/create" \
-u {API_KEY}: \
-d "size=300" \
-d "data=hello+world"
Response
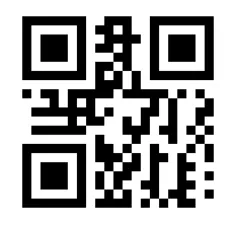
Merge PDF files
Merge multiple PDF files into a single file.
Request
curl "https://api-us1.stannp.com/v1/pdf/merge" \
-u {API_KEY}: \
-d "files[0]=https://file1.pdf" \
-d "files[1]=https://file2.pdf"
Response
{ "success": true, "data": "https:\/\/merged-file.pdf" }
Get templates
Get the templates created on your account.
Request
curl "https://api-us1.stannp.com/v1/templates/list" \
-u {API_KEY}: \
Response
{ "success": true, "data": [ { "id": "0", "template_name": "Template name 1", "size": "US-letter", "duplex": "1" }, { "id": "1", "template_name": "Template name 2", "size": "A6", "duplex": "1" } ] }